Major Programming Paradigms Explained (with Examples)
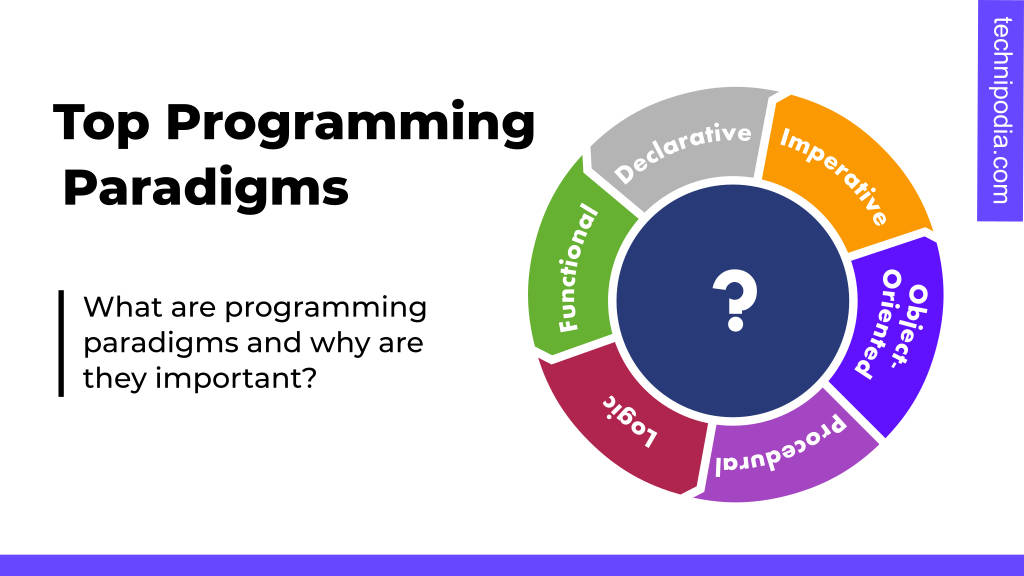
Early programming languages were not structured and this led to coders writing jumbled-up spaghetti code.
The programming languages that were developed later introduced rules and structures to make it easier to write organized code. This led to the development of programming paradigms.
In this article, I will look at:
- The most common programming paradigms.
- Examples of programming languages that use the different paradigms.
- Pros and cons of various programming paradigms.
By the end of the article, you should be able to understand which programming paradigms are used in the common programming languages and how they determine how these languages can be used.
What Is a Programming Paradigm?
A programming paradigm is a certain way or style of programming. It is a way of conceptualizing how to structure and organize code. [1] [2]
Why Are Programming Paradigms Important?
Programming paradigms:
- Provide rules and guidance on how to write code.
- Help us to organize and structure code.
- Help us to match a particular programming language to a particular task based on pros and cons. This is especially important when dealing with other people’s code, libraries, or frameworks.
- Help us to classify programming programs (see Types of Programming Languages)
- Help us to learn new programming languages. If you understand the programming paradigm used in a particular programming language, you can quickly pick up a related language.
Multi-Paradigm Programming Languages
Every programming language follows a particular programming paradigm. Some adhere to a specific programming paradigm while others are “multi-paradigm” languages, meaning that they allow the implementation of more than one programming paradigm. [4]
Examples of multi-paradigm programming languages include Python, JavaScript, Ruby and PHP among many others. [19]
In practice, you might end up working with several programming paradigms and most modern programming languages allow you to do that.
Top Programming Paradigms
There are many programming paradigms around. Some estimates put the number as high as 27 but the commonly used ones are few. Also, you can group or classify these paradigms in different ways. [1] [3]
In this article, for the sake of simplicity, I will explore the 6 major programming paradigms which will be grouped into two main types.[1]
- Imperative Programming Paradigm (with Procedural and Object-oriented programming paradigms).
- Declarative Programming Paradigm (with functional and logic programming paradigms).
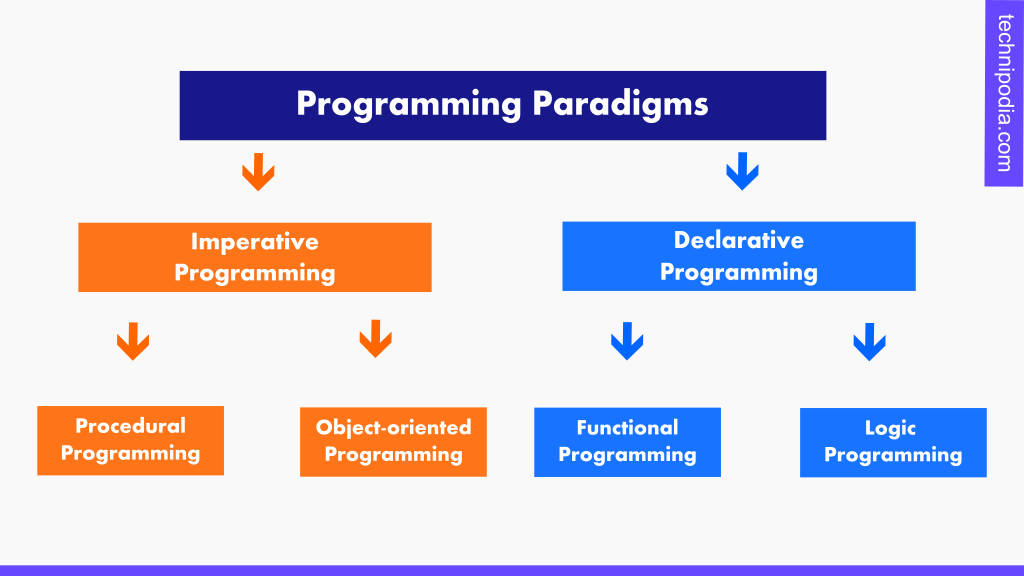
1. Imperative Programming Paradigm
In imperative programming, you give detailed instructions on how to achieve a particular result in a step-by-step manner. The name imperative comes from the Latin word ‘imperare’ which means ‘to command’. [5]
Imperative programming is explicit in that the commands show how to achieve the desired result.
An example in the real world is this. When giving directions to your house, you may say drive for 1km then turn left and drive for another 2km and stop at the blue gate.
Several programming paradigms fit under imperative programming including procedural and object-oriented programming. [1]
>> Example of Imperative Programming in Code
Here is an example of adding two numbers in Python that follows the imperative style. [17]
# A program for adding two numbers in Python num1 = 1.5 num2 = 6.3 # Add two numbers sum = num1 + num2 # Display the sum print('The sum of {0} and {1} is {2}'.format(num1, num2, sum))
>> Languages that use imperative programming
The best-known examples of imperative programming languages include C, C#, C++, FORTRAN, Java, COBOL, Python, and Ruby among others. [7]
>> Pros and Cons of Imperative Programming
Imperative programming has several pros and cons. [5]
Advantages of Imperative Programming | Disadvantages of Imperative Programming |
The code is easy to read and understand. | The code can be extensive and wordy. |
It is easy to learn this style of programming (i.e. it is beginner friendly). | For large complex programs, it can get confusing, especially if dealing with someone else’s code. Debugging can be a pain. |
It is widely used and there are plenty of learning resources and support | It can be difficult to optimize or extend code. |
#1. Procedural Programming
Procedural programming is an imperative style of programming based on procedure calls where the program is divided into a smaller set of instructions called procedures. Procedures are also known as subroutines, functions, or methods. [8]
Dividing programs into subroutines makes them more modular and easier to organize.
>> Features and benefits of procedural programming
Here are some of the distinctive procedural programming features, and the advantages and disadvantages they confer. [8] [9]
- It follows an imperative style where detailed instructions are given in a step-by-step manner. This makes the code easier to understand and follow.
- Program code is organized as a set of procedures or functions. A function is a block of code that contains a list of instructions to be carried out by the computer. Functions help to break down large projects into smaller chunks and this makes debugging easier.
- Functions can be reused in other parts of the program. This shortens the amount of code you need to write.
- Uses a top-down approach where a complex algorithm is broken down into smaller fragments which are then developed further. This makes it easier to track the program flow. However, it might lead to redundancy once you start to build up each fragment separately.
- Data and functions are detached from each other. This might be easier to implement in projects that are not complex.
>> Languages that use procedural programming
The best known examples of languages that use procedural programming include COBOL, FORTRAN, Pascal, ALGOL, BASIC, C, C++, and Visual Basic among others. [7] However, most modern languages support this style of programming.
>> Where is procedural programming used?
Below are some instances where procedural programming languages (such as C) have been used. [10]
- Creating operating systems. For example, the Linux Kernel is written in C.
- Creating embedded systems drivers and applications (C, C++).
- Creating database applications such as MySQL and Oracle.
>> Recommended resource for learning procedural programming
There are many programming languages that you can use to get started with procedural programming. One of the best languages for this is Python. It has become the most popular programming language to learn because of its application in Data Science and Artificial Intelligence (see Why Learn Python for more)
A good course for learning procedural programming is the Introduction to Python Programming certificate course from Georgia Tech University (available on Edx).
#2. Object-Oriented Programming
Object-oriented programming (OOP) is an imperative style of programming that is based on the concept of objects.
An object is an entity that contains attributes and functions. For example, if you have an object ‘Car’, it can have attributes like color, model, and size, as well as functions like drive, brake, and start. [9]
>> Features and benefits of object-oriented programming
Here are some distinctive features of object-oriented programming and the advantages they bring. [8] [9]
- Data (attributes) and functions (methods) are bound together in objects (i.e. encapsulation). Objects simplify the complex human world in ways we can understand. This makes troubleshooting and collaboration easier. It also protects data from unintended corruption.
- Only essential details are exposed to the user and the rest are hidden (i.e. abstraction). This helps to deal with complexity, especially for large projects.
- Child classes can inherit data and behaviors from one or more parent classes (i.e. inheritance). This helps developers to reuse common code and cut down on development time.
- Variables, functions, or objects can take on multiple forms (i.e. polymorphism). A single function, for example, can adapt to the class that it is placed in. This reduces complexity and the amount of code you have to write.
>> Examples of programming languages that use object-oriented programming
OOP is one of the most popular programming paradigms and it is present in many programming languages. These languages include Java, C++, Python, Javascript, PHP, R, and Scala among many others. [7] [9]
>> Where is object-oriented programming used?
OOP has many applications and it is especially useful in modeling real-world problems. It can be used in developing web and desktop applications, expert systems, office automation, object-oriented databases, and neural networks among many other uses. Its popularity stems from the fact that it is easier to write when creating complex programs. [11]
>> Recommended resource for learning object-oriented programming
You can learn OOP by studying any of the popular programming languages such as Java. Java has consistently ranked as one of the most popular programming languages for many years (see why in my article on Top Programming languages).
To get started with object-oriented programming check out the Introduction to Object-Oriented Programming with Java certificate course from Georgia Tech University (Available on Edx).
2. Declarative Programming Paradigm
In declarative programming, the primary focus is on describing the result that we want rather than specifying how we get that result in a step-by-step manner. [6]
Declarative programming is implicit in that the commands specify what the result should look like but not how to achieve it.
In a real-world example, when directing someone to your house, you give them your address and let them figure out how to get to your house.
Several programming paradigms fit under declarative programming including functional and logical programming. [1]
>> Example of Declarative Programming in Code
Here is an example of a program in Prolog for finding the maximum of two numbers and the minimum of two numbers. [18]
find_max(X, Y, X) :- X >= Y, !. find_max(X, Y, Y) :- X < Y. find_min(X, Y, X) :- X =< Y, !. find_min(X, Y, Y) :- X > Y.
>> Languages that use declarative programming
The best-known examples of declarative programming languages include Prolog, Lisp, Haskell, Erlang, Mercury, and SQL. [7]
>> Pros and Cons of Declarative Programming
Declarative programming has several advantages and disadvantages. [6]
Advantages of Declarative Programming | Disadvantages of Declarative Programming |
You can write less code compared to imperative programming. | Can be difficult to understand for someone not familiar with your code |
Programs can be written much quicker because you need less code. | Harder to learn compared to imperative programming |
Code is easier to optimize and extend |
#1. Functional Programming
Functional programming is a declarative style of programming where software is created using pure functions.
A pure function is a function that always returns the same result when you pass the same arguments. [12] [13]
>> Features and benefits of functional programming
Here are some key features of functional programming and the advantages they have. [12] [13]
- Pure functions make your code predictable and easy to understand because they don’t change any states and produce no side effects. This makes it easier to debug and write error-free codes.
- Functions are treated as any other variable (i.e. are first-class functions) and can be passed as an argument in another function, returned by another function, or can be stored as a value in a variable. This means you can write code that is short and clear.
- Since pure functions don’t modify variables or any data outside of the function, you can implement parallel programming.
- Functions can be tested as independent units and can also be reused in multiple places.
>> Examples of Programming Languages that use Functional Programming
Examples of purely functional languages include: Haskell, Elm, and PureScript [7]
Some programming languages are not purely functional but they support functional programming. Examples of these ‘impure’ functional languages include Python, Scala, Elixr, and Rust, among many others. [7]
>> Where is functional programming used?
Although functional programming has been around for over 60 years, it has gained popularity in recent times due to emerging trends in data science, parallel computing, and machine learning.
Functional programming has been prominently used where concurrency and parallelism are required. Erlang, for example, has been used at Ericsson for telecommunications. It was also used to create WhatsApp, a messaging app. [13]
>> Recommended resource for learning functional programming
A great way to get started with functional programming is to learn functional programming in Scala. Scala has become popular in recent years because of its applications in Data Science and Data Engineering. Big data tools like Apache Spark and Hadoop use Scala.
To get started with functional programming using Scala, check out the Functional programming using Scala course on Coursera.
#2. Logic Programming
Logic programming is a declarative style of programming that is based on formal logic rules where statements express facts and rules about problems within a system of formal logic. [12] [14] [15]
>> Features and benefits of logic programming
Here are some key features of logic programming and the advantages they have. [12] [16]
- Since the programs are based on formal logic rules, they are easier to understand and debug. This reduces maintenance costs because you can easily understand code written by other developers.
- Simpler algorithms and code result in faster and more efficient coding.
- Coding is efficient for problems that require inference. Complicated ideas can therefore be represented in code.
- It has good pattern matching and data searching capabilities.
- Lists and recursion are handled naturally.
>> Examples of Programming Languages that use logic programming
Prolog is probably the best-known example of a programming language that uses logic programming. Datalog and ASP are also popular. [7]
>> Where is logic programming used?
Logic programming languages are well suited to situations when you need to translate logic into code, for example in expert systems, artificial intelligence, fault diagnosis, natural language processing, and machine learning.[15]
>> Recommended resource for learning logic programming
If you are interested in Artificial Intelligence (A.I) and developing expert systems, Prolog is a great language to add to your skill set.
To get started in logic programming, check out the Prolog Zero to Hero course on Udemy.
Programming Paradigm FAQs
Here are some frequently asked questions about programming paradigms.
For a full list of the most interesting commonly asked questions about programming, see my article on Programming FAQs.
#1. Which are the most popular programming paradigms?
The most widely used programming paradigms are object-oriented and procedural programming. [2] Both use the imperative style of programming.
Languages using the imperative style of programming tend to dominate because this is how programming was done in the early days and it is how many people learned how to program.
These days, however, the declarative style of programming is becoming more popular especially as people become more dependent on libraries and frameworks.
#2. Which programming paradigm should you use?
There is no right or wrong programming paradigm. Some programming paradigms are better suited for some tasks than others. You need to choose a programming language that uses the programming paradigm that will best help you to achieve your desired result.
Many modern programming languages are multi-paradigm therefore you don’t have to worry too much about whether they support a particular paradigm.
#3. What is the difference between imperative and declarative programming?
Imperative programming gives explicit instructions on how to achieve the desired result. You need to give step-by-step instructions to the computer.
Declarative programming specifies the result that needs to be achieved but does not give step-by-step instructions on how to do it.
Wrapping Up
The topic of programming paradigms is broad and still evolving. In this article, I have provided a simple and broad overview of the topic.
However, there is still a lot more to learn about programming paradigms. If you want a simple, non-technical explanation of programming paradigms, you can check out the book A Review of Programming Paradigms through History on Amazon.
If you prefer video, check out the Paradigms of Computer Programming course from UCLouvain (on Edx).
Further Reading
If you are new to programming, you may also enjoy the following articles: